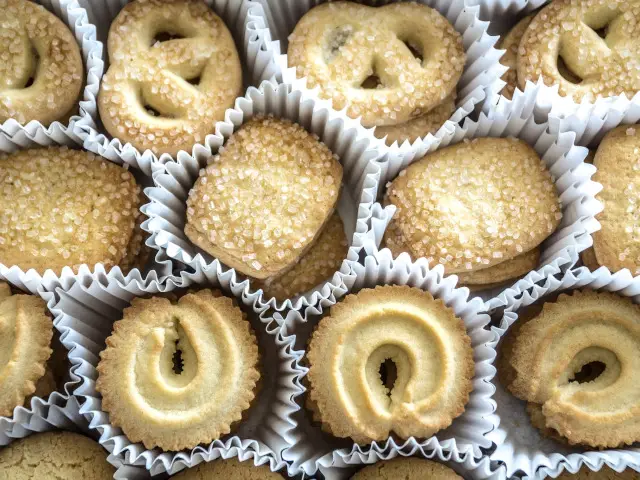
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
I started my CSS framework journey using Foundation but ultimately found it to be too bloated and inflexible for my needs. For several years after that I used my own lightweight float grid framework.
Times changed, and when I started using flexbox I decided that I was feeling too lazy to build my own framework again… so I started using Bulma. I like Bulma because it doesn’t force pre-built Javascript down my throat; I can switch between Javascript frameworks and still use my chosen CSS framework. It is also very flexibly coded in SASS, bonus!
So how do I include Bulma in my Nuxt projects?
You get the option to include Bulma with a fresh Nuxt install, but this isn’t actually particularly useful. Part of the appeal of using a framework written in SASS is the flexibility to change the variables. When loading Bulma this way all you get is the fixed CSS.
To change this we can make a change in the ‘nuxt.conf.js’ file in the root directory of our install. For those who aren’t familiar, it is the control centre for Nuxt. All of our settings here are reflected in the Nuxt generated Vue files. You can see where Bulma is loaded by navigating to .nuxt/app.js from the project root directory.
Back in the ‘nuxt.conf.js’ file, find the modules section and comment out the line ‘@nuxtjs/bulma’. When we rebuild our server instance we can now see that Bulma is no longer being loaded.
Right! Now we are in a position where Bulma is included in our project from the install but not being loaded by Nuxt. Our next step is to include the sass files instead of the css.
We can achieve this by adding the following to the ‘Global CSS’ section of the ‘nuxt.conf.js’ file:
css: [{ src: 'bulma/bulma.sass', lang: 'sass' }],
But by doing this all we are achieving is loading in the base sass to be compiled. It still isn’t going to give us access to the Bulma SASS variables.
We can give ourselves access to these variables by creating a separate stylesheet in our assets folder. I’ve called this ‘main.scss’. In this file we are going to add the following:
/* Import Bulma Utilities */
@import '~bulma/sass/utilities/initial-variables';
@import '~bulma/sass/utilities/functions';
/* Variable changes go here */
// Colours
$primary: #d0eeed;
$secondary: #d0e0ee;
// Breakpoints
$tablet: 724px;
$desktop: 960px + (2 * $gap);
$widescreen: 1152px + (2 * $gap);
$fullhd: 1344px + (2 * $gap);
/* Import the rest of Bulma */
@import '~bulma';
As you can see, we’ve imported the Bulma variables and functions, made the changes that we want to make and then imported Bulma framework. Now to let Nuxt know what to do with it.
Back in our ‘nuxt.conf.js’ file we overwrite the Global CSS import we created earlier and replace it with this:
css: [{ src: '~/assets/main.scss', lang: 'scss' }],
After rebuilding the server you should have full access to Bulma.
To install Bulma as a production dependency navigate to the root of your project install and type:
npm i -P bulma
Then follow the steps mentioned above.
Similarly to installing Bulma as a production dependency, we can do the same with Fontawesome:
npm i -P font-awesome
It has been kindly suggested in the comments that if you are having trouble building then you should make sure that you have the correct SASS dependencies installed.
npm install node-sass sass-loader -–save-dev
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!
You saved me after hours of struggle.
thank you
Thank you, I spent like 45 minutes trying to figure it out from other sources!
The final nuxt.config.js css configuration should look like:
css: [{ src: ‘~/assets/main.scss’, lang: ‘scss’ }],
“src” was missing.
Also, if your project is failing to build make sure you have the SASS development dependencies or else it can’t load the modules it needs to generate.
# npm install –save-dev node-sass sass-loader
Thank you! I’ve added your fix/suggestion to the post.
Great article thank you.
Has anyone else noticed you cannot use responsive breakpoints using this method? If I add +tablet it forces an error because of the mixins not being imported until `@import ‘~bulma’;`. However, if I include `mixins.sass`, I have to include `derived-variables.sass` as mixins refers to derived variables.
This results in the Bulma variables being more important than my overrides.
Bit of a pain…
Hi Grant
Try using the responsive mixins after the bulma import:
Hi Gav,
Thanks so much for this. Very helpful
Thanks, help me a lot.
Just a heads up I switched from LibSass (node-sass) to the new Dart Sass because I don’t like npm warning messages 🙂
So instead of running.
npm install node-sass sass-loader
Run the following.
npm install sass sass-loader
And everything worked perfectly.
Thank you for the tutorial.