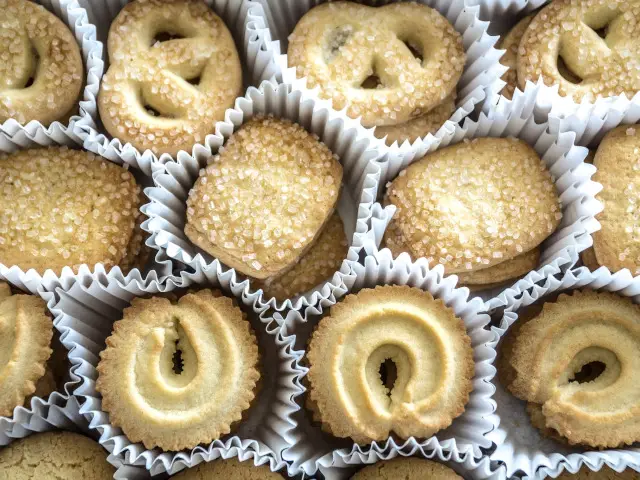
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Measuring code execution performance in JavaScript can help identify areas of your code that are slow or inefficient. This allows you the chance to optimise your code and ultimately produce a cleaner, more performant application.
Principally, to measure code execution we need to subtract the time the script starts from the time the script ends.
There are multiple methods for measuring code execution time in JavaScript. In this tutorial, we will explore several of these methods.
The console.time() method is simply a timer baked into the console object. We can call console.time() to start the timer and console.timeEnd() to stop. When the timer is stopped then the elapsed time is output to the console.
The console.time() method works using a string parameter to name the timer. In the examples I am using ‘gavsTimer’. Similarly, to end the timer and log the value you will have to pass the string name of a previously started timer.
console.time("gavsTimer");
// Put code you want to measure here
console.timeEnd("gavsTimer");
It is also worth noting that we can log the progress of the timer (without stopping it) using the console.timeLog() function. This function also takes a string parameter referencing a previously started timer.
console.time("gavsTimer");
// Put first part of code you want to measure here
console.timeLog("gavsTimer");
// Put second part of code you want to measure here
console.timeEnd("gavsTimer");
The console.timeLog() function is particularly useful if you are trying to snapshot several time intervals.
We can use the JavaScript Date() object to grab the current timestamp before and after the code we want to measure. Once we have those two values we only need to apply a simple sum to subtract the start time from the end time. The remaining value is the time taken to execute the time in between in milliseconds.
Using the Date() object has more similarities to the approach I demonstrated using PHP.
Note: I advise using Date.now() over Date.getTime() because it is around twice as fast and objectively semantically cleaner. This is largely because you are creating a new date object each time you use “new Date().getTime()”.
// Get the start time
const start = Date.now();
// Put code you want to measure here
// Get the end time
const end = Date.now();
// Subtract the start time from the end time to get the time taken
const elapsed = end - start;
console.log(`Script executed in ${elapsed} ms`);
The Performance.now() method uses high precision to return a high resolution timestamp in miliseconds. The difference between using this method and the date object is that Performance.now() is calculated relative to when the page was loaded, rather than relative to the Unix epoch (like with Date.now()).
Performance.now() also returns to a microsecond precision, but this precision comes with a large performance penalty. The rule of thumb should be, if you need a microsecond precision then use Performance.now(). If you don’t then use a different method.
// Get the high resolution start time
const start = performance.now();
// Put code you want to measure here
// Get the high resolution end time
const end = performance.now();
// Subtract the start time from the end time to get the time taken
const elapsed = end - start;
console.log(`Script executed in ${elapsed} ms`);
There are multiple ways to measure code execution performance in JavaScript, with the most popular options being the console.time() and console.timeEnd() methods, the Date() object, and the performance.now() method. Using these methods, you can identify performance bottlenecks in your code and optimize it for better performance.
For what it is worth, when I want to measure the performance of my JavaScript code then I use the Date() object approach.
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!