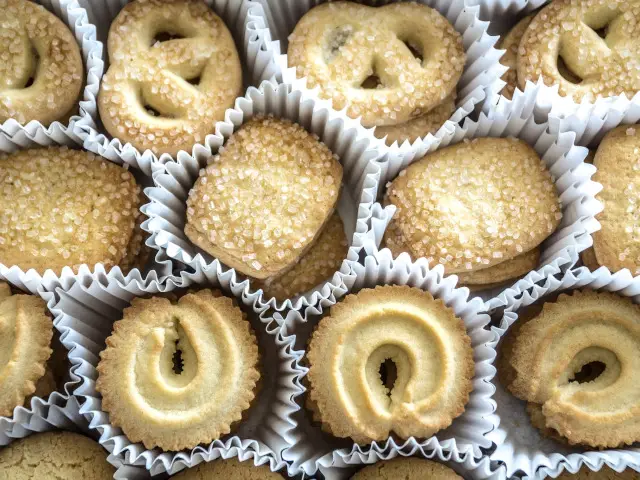
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Interestingly, I’d never really delved deeply into the comment functions of WordPress until I started my own blog. WordPress started life as a blogging CMS but almost all of the Websites I’ve built have been more ‘brochure’ focussed or contained ‘no-comment’ news blogs. I’m willing to guess that this is a common theme for most developers.
Let’s have a look at how to add comments to our custom and child themes.
Make sure that your theme has a comments.php file in the root directory. If it hasn’t then you’ll need to create one now.
This step is really easy. We start by checking whether the comments are allowed for the current post using comments_open(). If the condition is true we can load our comments template with the function comments_template(). By default this will return the comments.php from your current theme, but optionally you could pass the path to an alternative file with comments_template(‘/path-to-file.php’) .
Open up your post template and, identifying where you would like your comments to appear, paste the below code.
if (comments_open()){
comments_template();
}
At this stage it is unlikely that you have any comments to work with. Let’s make sure that you can create a comment.
We can do this by calling the WordPress comment form. Open up your comments.php and make sure that it is empty, then paste the code below.
comment_form();
As simple as that WordPress provides us with a default comment form. Submitted comments should now be available for us to query. Make sure to submit at least one.
Great, we’ve submitted a comment. Shame we can’t see it. We can check that the comment is definitely there by visiting the comments section of our WordPress admin but it would be much better if this displayed under our article.
Our comments.php is looking a little bit bare. Let’s fix that.
We are going to use a function called wp_list_comments() to loop through our comments. This uses a handy pre-built walker to display the tree structure of the comments. If the layout isn’t right for you then you can create your own walkers, but I’m not going to cover that in this article.
First we are going to double check that there are comments for us to display using the function have_comments().
if (have_comments()) :
endif;
Then we want to loop them into an ordered list using wp_list_comments().
if (have_comments()) : ?>
<ol class="post-comments">
<?php
wp_list_comments(array(
'style' => 'ol',
'short_ping' => true,
));
<?
</ol>
endif;
If you refresh your post then you should now see your comment. The styling isn’t great but I’ll leave that up to you to fix.
Note: Don’t forget to enqueue the reply script as this will allow users to reply to comments directly as well as the post. I do this in my global enqueue function. The following code should load the comment reply script into your footer.
if (is_singular() && comments_open() && (get_option('thread_comments') == 1)) {
wp_enqueue_script('comment-reply', 'wp-includes/js/comment-reply', array(), false, true);
}
The first thing I want to do is make a tweaks filter. This will allow us to customise the comment form. add the following to your functions.php.
function gb_comment_form_tweaks ($fields) {
return $fields;
}
add_filter('comment_form_fields', 'gb_comment_form_tweaks');
You may notice that the Name, Email and Website fields are below the comments text area. I’ve read that this is for usability reasons, but I personally don’t like it. If you would like to change that, like I have on Gav’s Blog, then use the code below.
//grab the comment
$comment_box = $fields['comment'];
//unset the array element
unset($fields['comment']);
//return the comment to the bottom of the array
$fields['comment'] = $comment_box;
Next we are going to add placeholder text to each field and remove the labels. You can actually change whatever you want at this point but I’m keeping most of the HTML the same.
$fields['author'] = '<input id="author" name="author" value="" placeholder="Name*" size="30" maxlength="245" required="required" type="text">';
$fields['email'] = '<input id="email" name="email" type="email" value="" placeholder="Email*" size="30" maxlength="100" aria-describedby="email-notes" required="required">';
$fields['comment'] = '<textarea id="comment" name="comment" cols="45" rows="8" maxlength="65525" placeholder="Comment*" required="required"></textarea>';
Your finished tweaks function should look something like the code below. Notice that I’ve tidied up by remaking the comments field and unsetting the url.
function gb_comment_form_tweaks ($fields) {
//add placeholders and remove labels
$fields['author'] = '<input id="author" name="author" value="" placeholder="Name*" size="30" maxlength="245" required="required" type="text">';
$fields['email'] = '<input id="email" name="email" type="email" value="" placeholder="Email*" size="30" maxlength="100" aria-describedby="email-notes" required="required">';
//unset comment so we can recreate it at the bottom
unset($fields['comment']);
$fields['comment'] = '<textarea id="comment" name="comment" cols="45" rows="8" maxlength="65525" placeholder="Comment*" required="required"></textarea>';
//remove website
unset($fields['url']);
return $fields;
}
add_filter('comment_form_fields', 'gb_comment_form_tweaks');
Finally I’ve revisited the comment_form() function by passing it an array to update the title and comment notes.
comment_form(array('title_reply' => 'Join the discussion!', 'comment_notes_before' => ''));
The final step is to adjust the comment settings in WordPress. You can find these on your admin panel under settings->discussion. Your requirements will likely differ to mine but I’ve decided to allow people to comment without making accounts and therefore have also disabled the avatar option.
I think my comments work quite nicely; they look good and are easy to use. The best part about it is that they were super easy to implement, as you can see. They don’t have to be fancy to be functional and WordPress actually offers some great customisation options out of the box.
For the future of Gav’s Blog comments I plan to explore a Vue.js alternative using the WordPress API. If you’re interested in a tutorial for this then please let me know!
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!
nice…
cool
nice
i need it.
Really good point
You don’t say.
Thanks much to you for such an awesome blog.
Thanks
Thanks
Thanks You
Love the article. I want to talk. Please contact me by email divingcyprus[at]gmail.com
You are a very clever person!
Go to the edit page for the post you want to disable comments on. Scroll down to the Discussion box, where you will find the comment options for that post. If you don t see a Discussion box, then click on Screen Options at the top of your screen, and make sure the Discussion checkbox is checked.
Here we go!
This might not be good on live production.
Article helped me, but you have a problem with the code – textarea is a pair tag, so when you don’t close it (which you don’t in the code), all the HTML after it will appear as text contents of the tag
Nice spot, thank you 🙂
Hello friend, code is working perfectly, but I want to know how to customize the display option of the comments. Means I want to add classes and css to the comments, just like you did in the comment form. please help
Thanks broo
just for testing ..
thank you for the quick tuto.
nice
That’s helpful. Thanks man..Although i am unable to understand previous comments.
Thank mate!
Now, this is absolutely clever of you. 🙂
Very usefull ty
Great
Cool
This might not be good on live production. Thanks for informative article.
Nice post.. it is very useful and informative
Great post, I feel its mandatory the comment section
Nice tutorial – I want to see how you do the thank you message when you submit
Hello Dear, Thanks for the sharing coding it’s really help full.
Nice article
Awesome. Im sure gonna apply this on my own planned blog
hello
Hi, Is there any way to auto trash spam comments on wordpress?
Great.
Its not working in my custom page template. Nothing showing on page. I am also trying to add comments_template() function but not worked.
haha
How can i add reply in comment form?
Thanks
This is awesome. Currently building my first WordPress blog theme. This was helpful.
dfhfdhb
testt
dfdf
gh
comment
Regular HVAC maintenance is the key to a seamlessly functioning system, ensuring efficiency, longevity, and a consistently comfortable indoor environment.
Good idea.
Nice Post
sss