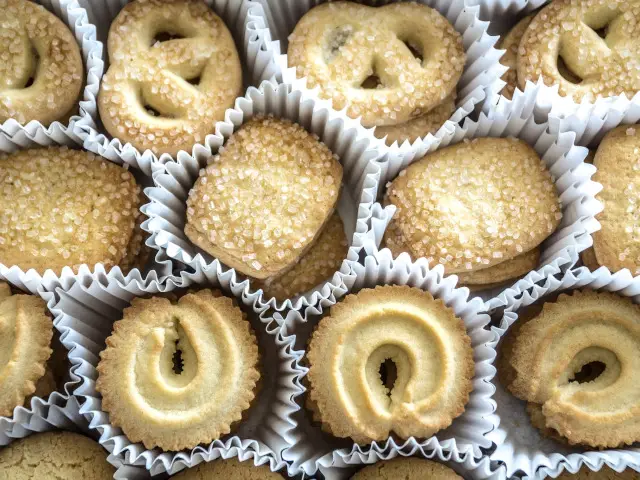
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
I’ve looked at how to find the closest number in a JavaScript array, but what happens when you simply want to check whether a value (or an array of values) exist within that array? Would you like to be able to pass an array to Includes()?
This post will explore how you can find out whether a JavaScript array contains certain values using simple functions to evaluate to true or false. If you want to skip straight to the end there are some ready to go ‘inArray’ style JavaScript functions for you to use.
Includes() is a simple array function which returns true if the passed value matches a value within the array.
let haystack = ["12345", "hello", "world"];
let needle = "world";
let result = haystack.includes(needle);
console.log(result); // Output = true
The problem with includes() is that it requires a string value and therefore you can’t pass an array to it. To ‘pass an array’ to includes() we have to try a different approach.
So includes() solves the problem of finding out whether a string exists within an array, but what happens if you want to match multiple string values? We could loop, map or filter the values to discover whether they exist, but the array prototype actually has a couple of very useful functions we can utilise.
The first of these is some(). This callback function will loop through an array until one of the conditions evaluates to true:
let haystack = ["12345", "hello", "world"];
let needle = ["world", "banana"];
let result = needle.some(i => haystack.includes(i));
console.log(result); // Output = true
To query the array to match all of the values it is very similar. In this instance we replace some() with every() which evaluates true if all of the conditions are met.
let haystack = ["12345", "hello", "world"];
let needle = ["hello", "12345"];
let result = needle.every(i => haystack.includes(i));
console.log(result); // Output = true
Finally, we can put these approaches together into a reusable function:
function inArray(needle, haystack, matchAll = false) {
if (matchAll) {
return needle.every(i => haystack.includes(i));
} else {
return needle.some(i => haystack.includes(i));
}
}
let result = inArray(needle, haystack, true);
Or if you like you could extend the array prototype. By doing this the function becomes available to all arrays. In this instance we are going to use the identifier ‘gb_’ to avoid possible code collisions from other libraries:
Array.prototype.gb_inArray = function(needle, matchAll = false) {
if (matchAll) {
return needle.every(i => this.includes(i));
} else {
return needle.some(i => this.includes(i));
}
};
let result = haystack.gb_inArray(needle, true);
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!
This post of yours saved me from hours of work. I was really struggling to get an answer for matching using every.
Thanks much.
What do you think about the following approach!
let hayStack = [“12345”, “hello”, “world”];
let needle = [“hello”, “12345”];
filterdData = hayStack.filter((item)=>{
return (
item.includes(‘hello’) &&
item.includes(‘1213’)&&
so on……..
)
})
Thanks mate!!
Hats off…brother.👏👏..big help..I just make a function for playing my wordle game by using your method.
make a functio