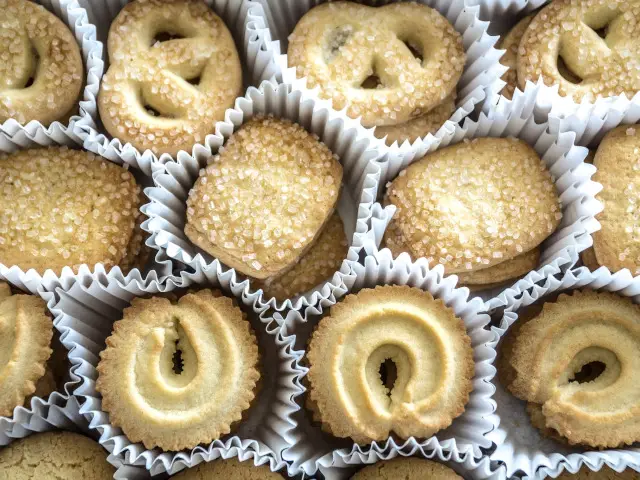
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
String interpolation is fancy ‘programmer’ talk for injecting variables into strings. Most programming languages have a way of doing this and we are going to have a look at JavaScript and Sass/scss in this post.
In JavaScript you are probably used to seeing strings handled like:
const variable1 = 'hello';
const variable2 = 'world';
console.log(variable1 + " " + variable2); // Output: 'hello world'
In ES6 we can use interpolation to make the code more human readable. The above example becomes something like this:
console.log(`${variable1} ${variable2}`); // Output: 'hello world'
Sass is no different to this and it actually becomes fundamental to use interpolation if we want to work with CSS variables.
body {
background-color: var(--some-css-variable, blue);
}
$colour: #fff;
body {
--some-css-variable: #{$colour};
}
This works in the same way if we want to use Sass variables inside of strings:
$themeColour: "red";
body {
background-color: blue;
&[theme="#{$themeColour}"] {
background-color: red;
}
}
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!