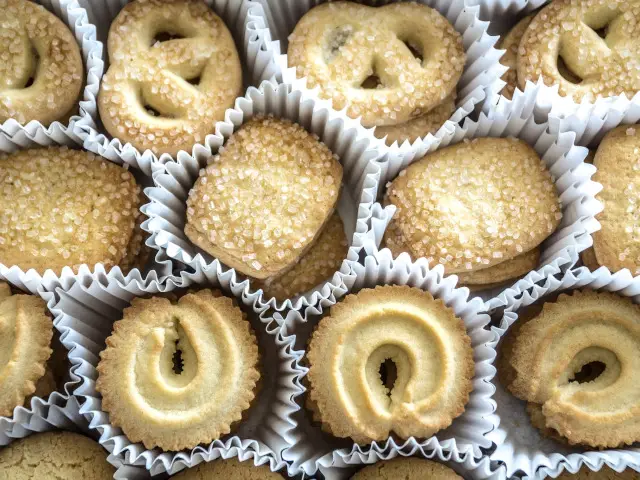
Create, register and use shortcodes in WordPress
18/03/2023Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
If you read my WordPress posts you will often see me ask you to enqueue a script or style sheet. This is bread and butter for WordPress theme and plugin development. It is how we call JavaScript scripts and CSS style sheets into our WordPress applications so you will need to know this for every theme you create, and the vast majority of plugins, too.
Don’t worry if you are unsure about how to do this, I’m going to show you.
Resources are enqueued through your theme or plugin functions.php file. We start by writing a function to handle our enqueues as a group.
function gb_scripts() {
}
We then call the script through an action with a low priority. This will cause it to load further down the page.
function gb_scripts() {
}
add_action('wp_enqueue_scripts', 'gb_scripts', 999);
Now that we have a function ready we can start by enqueuing a style sheet. We do this using the wp_enqueue_style() function. Here is how I call the main style sheet for Gav’s Blog.
wp_enqueue_style('gb-css', get_template_directory_uri() . '/css/style.min.css', array(), '', 'all');
Let’s break this down a bit:
Enqueuing scripts work roughly the same way as enqueuing style sheets. We use the wp_enqueue_script() function for this.
wp_enqueue_script('gb-js', get_template_directory_uri() . '/js/scripts.js', array( 'jquery' ), '', true);
Here is the code breakdown:
A basic theme enqueue function will look something like this:
Note: I’ve included a Google Fonts enqueue, too.
function gb_scripts() {
// Adding scripts file in the footer
wp_enqueue_script('gb-js', get_template_directory_uri() . '/js/scripts.js', array( 'jquery' ), '', true);
// Register main stylesheet
wp_enqueue_style('gb-css', get_template_directory_uri() . '/css/style.min.css', array(), '', 'all');
// Register Open Sans Google Fonts
wp_enqueue_style('open-sans-google-font', 'https://fonts.googleapis.com/css?family=Open+Sans', false);
}
add_action('wp_enqueue_scripts', 'gb_scripts', 999);
Sometimes you might like to include a resource on a specific post or template. For me I find this useful when I only need a resource for a specific article.
A good example of where everybody might be using this can be seen on my comments guide.
if (is_singular() && comments_open() && (get_option('thread_comments') == 1)) {
wp_enqueue_script('comment-reply', 'wp-includes/js/comment-reply', array(), false, true);
}
As you can see, I wrap the enqueue in an if statement so that my comment-reply script is only used when we are viewing a post (singular), the comments are open and when threaded comments are enabled.
Learn how to create and register your own WordPress shortcodes to add dynamic content to your posts and pages.
Learn how to improve code readability and performance by using guard clauses in JavaScript. Discover their benefits and best practices.
Learn the difference between implements and extends in TypeScript. Use Implements to implement interfaces and types, and extends to inherit from classes.
In this tutorial we will look at using YAML in PHP. Learn about Parsing and Writing YAML files using Symfony's YAML component.
Measuring code execution performance is an important way to identify bottlenecks. Use these methods in JavaScript to help optimise your code.
Find bottlenecks, optimise and clean your code, and speed up your apps by measuring the execution time of your PHP scripts using microtime.
Learn how to regenerate and update WordPress media and image sizes both programmatically (without plugin), and also with a handy plugin.
Ever seen constants like __DIR__ and __FILE__ being used in PHP? These are 'Magic Constants', and this is how we can use them.
Learn how to use event listeners to detect and handle single and multiple keypress events in JavaScript. Add modifier keys to your application!